|
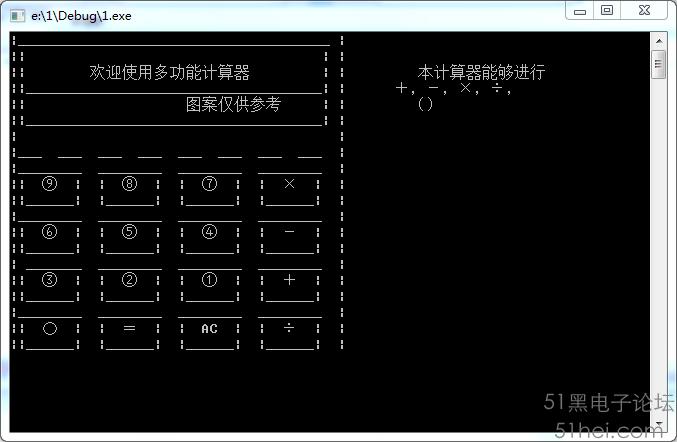
- #include<stdio.h>
- #include<conio.h>
- #include<math.h>
- #include<iostream>
- /*****************************************/
- /* 將數(shù)字字符轉(zhuǎn)化成浮點(diǎn)型實(shí)數(shù)進(jìn)行計(jì)算 */
- /* */
- /*****************************************/
- double readnum(char f[],int*i)
- {
- double x=0.0;
- int k=0;
- while(f[*i]>='0'&&f[*i]<='9')
- {
- x=x*10+(f[*i]-'0');
- (*i)++;
- }
- if(f[*i]=='.')
- {
- (*i)++;
- while(f[*i]>='0'&&f[*i]<='9')
- {
- x=x*10+(f[*i]-'0');
- (*i)++;
- k++;
- }
- }
- while(k-->0)
- {
- x=x/10.0;
- }
- return (x);
- }
- /*******************************/
- /* 計(jì)算后綴表達(dá)式的值 */
- /*******************************/
- double evalpost(char f[])
- {
- double obst[10];
- int top=0;
- int i=0;
- double x1,x2;
- while(f[i]!='=')
- {
- if(f[i]>='0'&&f[i]<='9')
- { obst[top]=readnum(f,&i);top++;}
- else if(f[i]==' ')
- i++;
- else if(f[i]=='+')
- {
- x1=obst[--top];
- x2=obst[--top];
- obst[top]=x1+x2;
- i++;
- top++;
- }
- else if(f[i]=='-')
- {
- x1=obst[--top];
- x2=obst[--top];
- obst[top]=x2-x1;
- i++;
- top++;
- }
- else if(f[i]=='*')
- {
- x1=obst[--top];
- x2=obst[--top];
- obst[top]=x1*x2;
- i++;
- top++;
- }
- else if(f[i]=='/')
- {
- x1=obst[--top];
- x2=obst[--top];
- obst[top]=x2/x1;
- i++;
- top++;
- }
- }
- return obst[0];
- }
- /***********************************/
- /* 判斷字符是否為操作字符 */
- /***********************************/
- int is_operation(char op)
- {
- switch(op)
- {
- case'^':
- case'K':
- case'+':
- case'-':
- case'*':
- case'/': return 1;
- default: return 0;
- }
- }
- /*****************************/
- /* 判斷字符的優(yōu)先級 */
- /*****************************/
- int priority(char op)
- {
- switch(op)
- {
- case'=': return -1;
- case'(': return 0;
- case'+':
- case'-': return 1;
- case'*':
- case'/': return 2;
- default: return -1;
- }
- }
- /******************************/
- /* 中綴表達(dá)式轉(zhuǎn)化成后綴表達(dá)式*/
- /******************************/
- void postfix(char e[],char f[])
- {
- int i=0,j=0,k=0;
- char opst[100];
- int top=0;
- opst[0]='=';top++;
- while(e[i]!='=')
- {
- if((e[i]>='0'&&e[i]<='9')||e[i]=='.')
- f[j++]=e[i];
- else if(e[i]=='(')
- { opst[top]=e[i];top++;}
- else if(e[i]==')')
- {
- k=top-1;
- while(opst[k]!='(') {f[j++]=opst[--top];k=top-1;}
- top--;
- }
- else if(is_operation(e[i]))
- {
- f[j++]=' ';
- while(priority(opst[top-1])>=priority(e[i]))
- f[j++]=opst[--top];
- opst[top]=e[i];
- top++;
- }
- i++;
- }
- while(top) f[j++]=opst[--top];f[j]='\0';
- }
- void print_1()
- { printf("|_______________________________________ | \n");
- printf("|| | | \n");
- printf("|| 歡迎使用多功能計(jì)算器 | | 本計(jì)算器能夠進(jìn)行 \n");
- printf("||_____________________________________| | +,-,×,÷,\n");
- printf("|| 圖案僅供參考 | | () \n");
- printf("||_____________________________________| | \n");
- printf("| | \n");
- printf("|___ ___ ___ ___ ___ ___ ___ ___ | \n");
- printf("|________ ________ ________ ________ | \n");
- printf("|| ⑨ | | ⑧ | | ⑦ | | × | | \n");
- printf("||______| |______| |______| |______| | \n");
- printf("|________ ________ ________ ________ | \n");
- printf("|| ⑥ | | ⑤ | | ④ | | - | | \n");
- printf("||______| |______| |______| |______| | \n");
- printf("| _______ ________ ________ ________ | \n");
- printf("|| ③ | | ② | | ① | | + | | \n");
- printf("||______| |______| |______| |______| | \n");
- printf("|________ ________ ________ ________ | \n");
- printf("|| 〇 | | = | | AC | | ÷ | | \n");
- printf("||______| |______| |______| |______| | \n");
- getch();
- system("cls");
- }
- void printf_2()
- {system("cls");
- printf("\n\n\n\n\n\n\n\n\t\t\t ##############################\n");
- printf("\t\t\t # #\n");
- printf("\t\t\t #----------謝謝使用----------#\n");
- printf("\t\t\t # #\n");
- printf("\t\t\t ##############################\n");
- printf("\t\t\t --XXXXXX制作\n ");
- }
- /****************/
- /* 轉(zhuǎn)化 */
- /****************/
- void zhuanhuan(char g[],char e[])
- {
- int k,i,j=0;
- for(i=0;g[i]!='=';i++)
- {
- k=i+1;
- if(g[i]=='('&&g[k]=='-')
- {
- e[j++]=g[i];
- e[j++]='0';
- }
- else e[j++]=g[i];
- }
- e[j]='=';
- }
- int main()
- {
- int wei;
- char e[100],f[100],g[100];
- int sign;int flag;
- print_1();
- do
- {
- printf("輸入所要經(jīng)計(jì)算的表達(dá)式(如:a*b/(c-d)=):\n");
- scanf("%s",g);
- zhuanhuan(g,e);
- postfix(e,f);
- printf("輸出保留幾位小數(shù):\n");
- scanf("%d",&wei);
- printf("%.*lf\n",wei,evalpost(f));
- while(1)
- { flag=3 ;
- printf("繼續(xù)計(jì)算/退出?1/0?");
- sign=getch();
- printf("%c\n",sign);
- switch(sign)
- {
- case '1':flag=1;getch();break;
- case '0':flag=0;getch();break;
- default: printf("非法輸入,請重新輸入:\n");
- }
- if(flag==1||flag==0)break;
- }
- }while(flag==1);
- printf_2();
- return 0;
- }
復(fù)制代碼
|
|